Looking to display sunrise or sunset times in your website using Javascript? While there are plenty of ways to do this including using the SunCalc library to calculates times on the fly in this quick guide I am going to show you how to use the free SunriseSunset.io API.
Full disclosure: I made this free JSON API to simplify the process of getting sunrise and sunset times in applications and web apps.
The best thing about the API is that it localizes the times to the area you are querying. This is actually why I initially built the API, I noticed many other sunset time APIs made finding proper times for locations complex. Using the default settings in SunriseSunset.io you’ll be returned with times at the specific location.
Using the SunriseSunset.io API
Using the free JSON API from SunriseSunset.io is super simple. All you need to do is put in a latitude and longitude and you’ll instantly retrieve sunrise/sunset times for that location. As you can see in the example below.
https://api.sunrisesunset.io/json?lat=38.907192&lng=-77.036873
The API doesn’t require any API key or authentication.
The API also includes additional options for setting the timezone or looking up a specific date. For specifics of additional parameters I recommend reading the documentation.
You can get coordinates for any locations on Google Maps simply by right clicking a spot on the map.
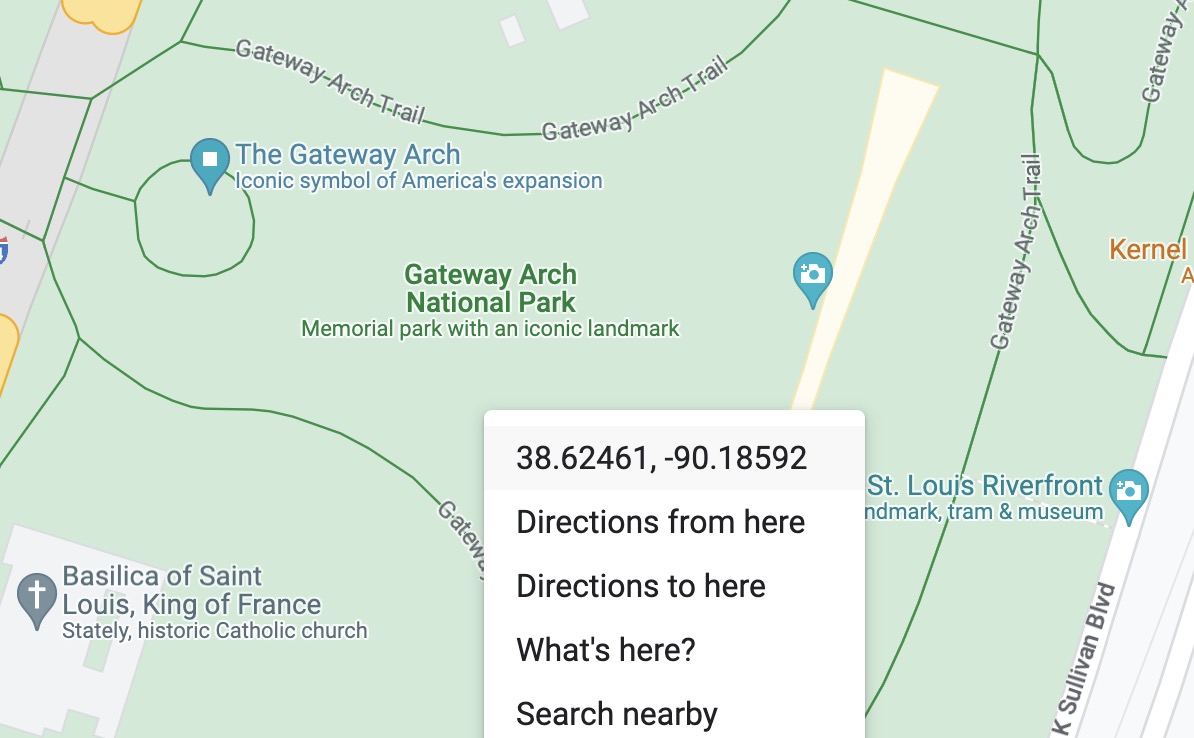
Here is an example using the coordinates of the Gateway Arch in St. Louis. This Javascript queries the SunriseSunset.io API and returns the resulting data in the console log. Of course you can use the data any way you please, like showing it in a dashboard or just using it in another function.
// Coordinates for Gateway Arch in St. Louis
const latitude = 38.624519738607894
const longitude = -90.18581515692256
const url = `https://api.sunrisesunset.io/json?lat=${latitude}&lng=${longitude}`
fetch(url)
.then(response => response.json())
.then(data => {
console.log(data)
})
.catch(error => console.error('Error:', error))
Here is a more flushed out example on CodePen that actually shows displaying the sunrise and sunset times on the page and the raw output of the API.
Conclusion
In conclusion, the JSON Sunrise and Sunset Times API on SunriseSunset.io is a powerful tool that can be used to enhance the functionality of your website or application.
Some example usage may include creating a tool for photographers, showing sunset times for a specific location like a business, or just using a user’s location and telling them the sunset times.
By providing accurate and up-to-date information on the sunrise and sunset times for any location on Earth, this API can help you create a more engaging and informative user experience for your visitors.